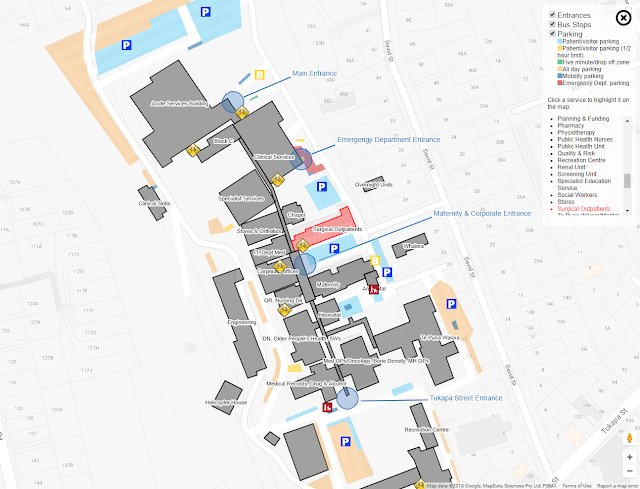
Clicking a building will show details:
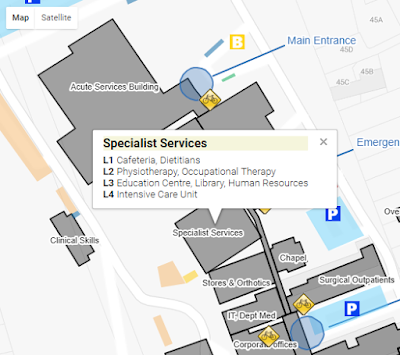
Doing whatever:
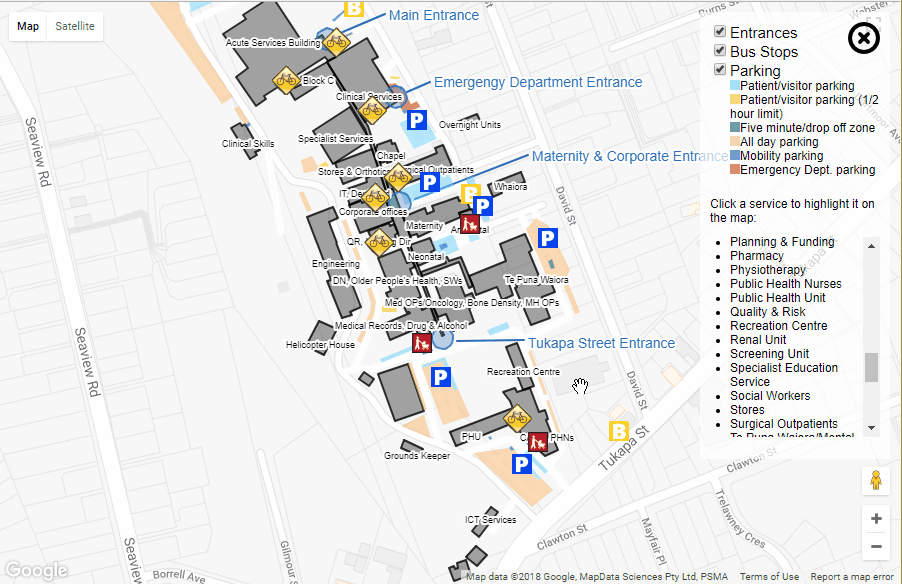
'Ambulance house' and 'Helicopter house' may or may not be the offical names of the buildings.
var buildings = [];
var ict = {
title: "ICT Services",
key: "itServices",
titleCoordinates: { lat: -39.0747770079001, lng: 174.0569919347763 },
description: "Applications, Business Intellignence, Reporting and Development",
coordinates: [{
lat: -39.07470621091739,
lng: 174.05701607465744
}, {
lat: -39.07475202073784,
lng: 174.0570965409279
}, {
lat: -39.074962329077316,
lng: 174.05688732862473
}, {
lat: -39.07491027261602,
lng: 174.0568122267723
}]
}
buildings.push(ict);
etc - add more buildings as required
This mob of co-ordinates is just referenced in the HTML
<script type="text/javascript" src="buildingPersistance.js"></script>
<script type="text/javascript" src="otherPersistance.js"></script>
Ok.
You have to sign up with google api and get a map key. Cram the script tag in your html:
<script src="https://maps.googleapis.com/maps/api/js?key=[your key here]"></script>
Hook up an initialisation method to run when the maps stuff is loaded
google.maps.event.addDomListener(window, 'load', initMap);
You need an initialisation method
function initMap() {
var uluru = {
lat: -39.072474,
lng: 174.055992
};
map = new google.maps.Map(document.getElementById('map'), {
center: uluru,
zoom: 17,
styles: [{
featureType: 'poi',
stylers: [{
visibility: 'off'
}]
}, {
featureType: 'transit',
elementType: 'labels.icon',
stylers: [{
visibility: 'off'
}]
}]
});
// draw any buildings we have
drawBuildings(map, buildings);
etc ...
When creating the map object the center will set the start location of the map
Styles defines what objects you want to show on the map (a heap of stuff shows by default)
Draw the things you want
function drawBuildings(map, buildings) {
for (var i = 0; i < buildings.length; i++) {
var building = buildings[i];
var buildingCoords = [];
for (var c = 0; c < building.coordinates.length; c++) {
var coordinate = building.coordinates[c];
buildingCoords.push(new google.maps.LatLng(coordinate.lat, coordinate.lng));
}
var strokeColor = building.strokeColor ? building.strokeColor : '#000';
var fillColor = building.fillColor ? building.fillColor : '#000';
var buildingDefinition = new google.maps.Polygon({
paths: buildingCoords,
draggable: false,
editable: false,
strokeColor: strokeColor,
strokeOpacity: 0.8,
strokeWeight: 2,
fillColor: fillColor,
fillOpacity: 0.35,
originalStrokeColor: strokeColor,
originalFillColor: fillColor,
key: building.key,
description: building.description,
title: building.title
});
buildingDefinition.setMap(map);
Adding a popup for a building
// build the popup information if we have a description for this building
if (buildingDefinition.description) {
buildingDefinition.addListener('click', function(event) {
var contentString = '<div class="info-list-title">' + this.title + "</div>" + this.description;
infowindow.setContent(contentString);
infowindow.setPosition(event.latLng);
infowindow.open(map);
});
}
Creating a label for a building (using maplabel-compiled.js from ... somewhere?)
// create a label for this building
if (building.title && building.titleCoordinates) {
var mapLabel = new MapLabel({
text: building.title,
position: new google.maps.LatLng(building.titleCoordinates.lat, building.titleCoordinates.lng),
map: map,
fontSize: 9
});
mapLabels.push(mapLabel);
}
No comments:
Post a Comment